Opengl Snake Game Source Code
List of class that I have made are:-
loadBMP.h //file use for loading bitmap image for texture map
class Image {
public:
Image(char* ps, int w, int h);
~Image();
char* pixels;
int width;
int height;
};
Image* loadBMP(const char* filename);
//Reads a bitmap image from file.
drawing.h //all image drawing for game content in this file
#include <glut.h>
#include <iostream>
//#include <stdio.h>
//#include <math.h>
#include 'loadBMP.h'
class Ball; //creating object for class ball
typedef struct
{
int X;
int Y;
int Z;
double U;
double V;
}VERTICES;
class drawing
{
private:
Ball *ball;
GLuint _cellingtextureID;
GLuint _walltextureID;
GLuint _floortextureID;
GLuint _secondfloortextureID;
GLuint _cubetextureID;
GLuint _gatetextureID;
GLuint _balltextureID;
GLuint _poletextureID;
GLuint _foodtextureID;
//GLuint _gatetextureID;
GLint CELL;
GLint poleDisplayList;
GLint gateDisplayList;
GLint roomDisplayList;
GLint cubeDisplayList;
GLint foodDisplayList;
GLuint menuDisplayList;
/*const*/ int space;// = 10;
/*const*/ int VertexCount;// = (90 / space) * (360 / space) * 4;
VERTICES *VERTEX;//[VertexCount];
int PI;
GLfloat CELL2;
GLfloat CUBE_POS;
GLfloat FOOD_POS;
int cube_ran_pos[6][6];
int food_ran_pos[4][4];
bool Gamestart;
void startwindow();
void drawRoom();
void drawBall();
void setupPole();
void setupGate();
void drawcube();
void drawFood();
void DisplaySphere(double);
//void drawGate();
void drawPole_and_Gate();
void setupLighting();
//int random_num(int);
public:
drawing();
void CreateSphere (double R, double H, double K, double Z);
void callBallConstructor();
//void setdrawing();
void draw();
~drawing();
void checkleftkey();
void checkrightkey();
void checkupkey();
void checkdownkey();
};
//this class used to draw image
game.h //this file content ball class from where all ball action is controled
//#include<vector>
#include <glut.h>
//const float BARRIER_SIZE = 0.12f;
class Ball {
private:
//The radius of the ball
float r;
//The x coordinate of the ball
float x0;
//The y coordinate of the ball
float y0;
//The z coordinate of the ball
float z0;
//The angle at which the ball is traveling. An angle of 0 indicates the
//positive x direction, while an angle of PI / 2 indicates the positive
//z direction. The angle is always between 0 and 2 * PI.
float angle0;
//The fraction that the ball is 'faded in', from 0 to 1. It is not 1
//when the ball is fading in or out.
float fadeAmount0;
//Whether the ball is fading out
bool isFadingOut0;
//collision detection
void DetectCollision();
public:
//Constructs a new ball with the specified radius, x, y and z coordinates,
//and angle of travel. An angle of 0 indicates the positive x
//direction, while an angle of PI / 2 indicates the positive z
//direction. The angle must be between 0 and 2 * PI.
Ball();
//Returns the radius of the ball
float radius();
//Returns the x coordinate of the ball
float x();
//returns the z coordinate of the ball
float y();
//Returns the z coordinate of the ball
float z();
//Returns the angle at which the ball is traveling. An angle of 0
//indicates the positive x direction, while an angle of PI / 2 indicates
//the positive z direction. The returned angle is between 0 and 2 * PI.
float angle();
//Causes the ball to begin fading out
void fadeOut();
//Returns whether the ball is fading out
bool isFadingOut();
//sets the angle of ball
void setangle();
//checks whether the left key is pressed
void checkleftkey();
//checks whether the right key is pressed
void checkrightkey();
//checks whether the up key is currently depressed
void checkupkey();
//checks whether the down key is currently depressed
void checkdownkey();
};
I hope you guys got a little idea about this game. Download it's complete source code and compiled it in code block or visual studio but don't forget to set up GLUT(OpenGL).
Thank you.
Hi everyone ,
In this blogpost I would like to share my OpenGl game project which I had developed for my semester course on Computer Graphics . It took almost a week to complete this project .
Project Description
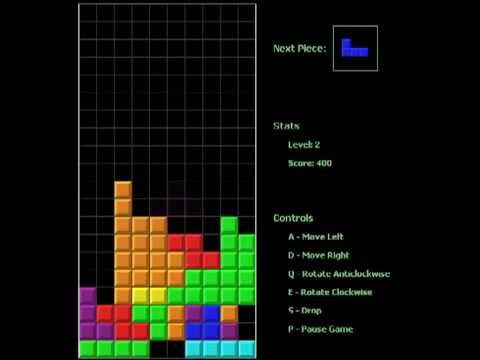
The project’s aim was to create a 2d snake game . This snake keeps on growing with the consumption of fruit . The game ends with the snake either colliding with itself or with the blocks . The game has a number of features associated with it like specular lighting effect, score and high score, play/pause the game and 3 exciting game levels :- worm, snake and kobra .
3d Snake Game Opengl Source Code
Keys
Hi guys, a beginner here. I've been trying to develop my own rendition of Snake using C and openGL. This is a 3D game. I have done everything from the eating of food, the 3D look, the snake's movement, EXCEPT, the growing of its tail. Source Code For Snake Game Using Opengl Codes and Scripts Downloads Free. Accounting source code for Delphi. This project is the source code for the article Java in 7 steps. Lugaru: The Rabbit's Foot is a 2005 video game developed and published by Wolfire Games. The game it's available on itch.io, Steam, and the developer's store. On May 11, 2010, Wolfire Games released the source code to the game under the GNU Public License Version 2 (or any later version). LibGDX is a cross-platform Java game development framework based on OpenGL (ES) that works on Windows, Linux, macOS, Android, your browser and iOS. LibGDX provides a well-tried and robust environment for rapid prototyping and fast iterations. LibGDX does not force a specific design or coding style on you; it rather gives you the freedom to create a game the way you prefer.
Opengl Snake Game Source Code Free
Up – moves up
Down – moves down
Left – moves left
Right – moves right
p/P – pauses game
any other key – resumes game
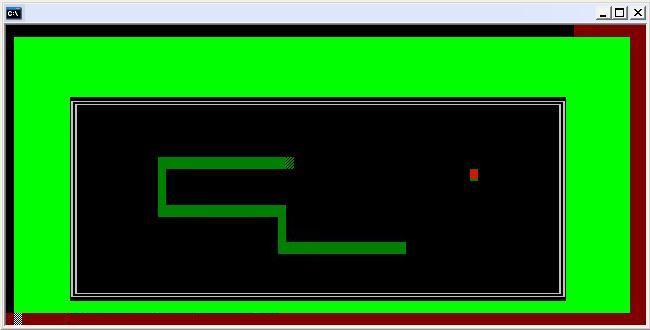
Platform :- Linux / Ubuntu
Opengl Snake Game Source Code Pdf
How to Run
Change the .doc extension to .zip
Unzip the file
run the file using ./snake in the terminal
compile the files using gl -o snake *.cpp
Opengl Snake Game Source Code Download
Source code can be downloaded from here :- snake